4.2. Primary Software Tools
Now that you have setup your Raspberry Pi 5, you will need to get familiar with a couple of software tools that will be used all throughout the project. This section will guide you through the installation and usage of all these primary tools and programs.
4.2.1. Terminal
The terminal prompt is a very powerful tool that we will be getting familiar with all along the project. At first it is very daunting but in a few months you will be navigating inside of it like a fish in the water. Once you discover the power it has, you might not even want to return to your classical screen!
Note
Software engineers refer to screens as GUIs for Graphical User Interfaces and to terminal as CLIs for Command Line Interfaces.
Any command in this documentation that is meant to be run inside a terminal will be inside square boxes written in an equally spaced font called a monospace font:
This is a command
Let’s play with a terminal! Turn on your Raspberry Pi, log into it and open a terminal by doing either:
CTRL-ALT-t
Windows key, search “terminal”, ENTER
The first command you will run is the classic “Hello, World!”. Copy the following line from the website and paste it into your terminal:
Warning
CTRL-v will not paste in a terminal, you need to do CTRL-SHFT-v or right click, paste!
echo "Hello, World!"
Commands
Here the command being run is echo
.
Arguments
echo
takes one argument that we wrap in apostrophes to denote
that it is one continuous string of text, it is bad practice but
you could also run:
echo Hello, World!
Flags
In addition to arguments, you can also pass flags
to echo
! Flags are little options used to tune the output of
our programs. Try out the -n flag:
echo -n "Hello, World!"
It suppresses the newline (\n) character that echo
adds by default
to wrap the following text on a different line to the one that you’re outputting.
Let’s try to add the newline (\n) character by hand ourselves:
echo -n "Hello, World! \n "
This doesn’t seem to work as it prints out the \n character in plain text instead of interpreting it as a newline.
Manual
Let’s check out the man page of the echo
command:
man echo
You see that in the description, it says:
-e enable interpretation of backslash escapes
Hint
Quit the man page by typing ‘q’
Every program in Linux has a man page so if you are lost, simply run:
man my-program
It will give you a detailed manual of how to use the program.
Let’s get back to echo
, we understood that adding the -e
flag enables the interpretation of backslash escapes so let’s try to add
that flag to our command:
echo -n -e "Hello, World! \n"
Now our \n newline character is correctly understood and it wraps the next line on a newline. Try adding 10 more \n and see what it does!
Comments
Comments in shell (shell is the programming language of the terminal) always start by a hashtag. Try to run the following comment in your terminal:
# Any line starting with a hashtag will be ignored by your terminal
It doesn’t do anything.
sudo
You might run into some commands that require admin rights to work.
To grant admin rights to the program your command is running, you need
to start the command with sudo
, try this following command:
dmesg
dmesg: read kernel buffer failed: Operation not permitted
Indeed, this command will list all the sensitive operations done by your kernel since the startup of your computer, now try:
sudo dmesg
It will ask you for a password, enter it.
Warning
When you type in password, your screen will stay blank as to protect your privacy (in case someone is looking behind your shoulder)!
Now you will see all the messages from your kernel, well done!
Conclusion
command
- the first word you type into your terminalargument
- mandatory things you pass to your commandsflags
- optional things you pass to your command (start with “-“)man ...
- the manual page of the command# ...
- comments in shellsudo ...
- super-user do, gives you admin privileges
Congratulations, you now know how to use a terminal!!
Here are some extra commands you will need to memorise for future work on the project:
pwd
- print working directory (shows you where you are)ls
- lists files in current directorycd dir
- changes directory to ‘dir’ directorycd ..
- goes up one directory (goes back)cd
- goes to home directory (~/)
With these basic commands you should be able to navigate around your linux system from a terminal like you’re used to doing it from the file explorer on your Windows PC or finder on a Mac.
4.2.2. nano
nano is a text editor, it is similar to programs you use to edit text such as:
Microsoft word
Notepad
In Linux there are countless options for text editors but we will start by learning basic text editors integrated inside the terminal such as nano as they will come in handy during the project.
nano is the simplest terminal text editor so we will learn it first.
Open a terminal and type cd
to go to the home directory ~/
.
Now run ls
to check what files are in this directory.
We will create a new file called ‘microver.txt’ by running:
touch microver.txt
Feel free to run ls
again to make sure that the file has really
appeared. If it’s all good, print out its contents to your terminal by
running:
cat microver.txt
As it is empty, it shouldn’t return anything.
Now let’s open it with nano:
nano microver.txt
Type some text in there and save your work with:
CTRL-o
It will prompt you to change the filename, if you want to keep it as is (microver.txt), simply press:
ENTER
Now exit the text editor by entering:
CTRL-x
Check that the content you put in the file is still there by printing out the contents of the file to your terminal again:
cat microver.txt
You should see the content you wrote in your file, well done! Now let’s delete the file from your computer:
rm microver.txt
Danger
Running rm
means remove forever, there is no trash can!
Congratulations, you now know how to create, edit and delete files right
from your terminal! (The next step is to learn vim
;) )
4.2.3. git
git is a version control program written by Linus Torvards (who also created Linux by the way).
Let’s install it to your device:
sudo apt install git -y
git can save your files locally or on a server. The most popular way to use git is by using the version hosted on github.com’s servers.
But why do we need to use a version control system?
You might find the sd card inside your Raspberry Pi dead one morning or worse, drop a screw on the circuit board and fry it in front of your eyes. If this was to happen, not only would you need to purchase a new Raspberry Pi but you would also need to rewrite every single line of code you have done until now. For this reason, you need to backup your code.
If you are alone, google drive is sufficient to backup your code but if you are 10 working on the same code files at the same time, you will have to save a copy of the codebase in a 10 different folders on the google drive, each called by the name of the person saving inside of them.
4.2.4. VSCode
4.2.5. Python3
Python3 is the main programming language we will be using to configure and control our rover, here is how to install it on your Raspberry Pi 5 !
Start by calling the apt
command to update your packages:
Note
apt
is the default package manager in Ubuntu
# Make sure your packages are up to date
sudo apt update
# Download and install new packages
sudo apt upgrade -y
Now install python3 on your system using apt
again:
# Install python3
sudo apt install python3 -y
Note
The -y flag here means ‘yes’ and avoids you having to confirm
You now have access to the python3 command which will run any python code you give to it. You can find where the apt package manager installed that python3 file by running:
which python3
This will probably return /usr/bin/python3
. This means that the
python3 executable is installed on your system and you can execute it no
matter which directory you are currently in.
Hint
bin/ directories are not the trash! They are where binary files are stored. Binary files are files made of 1s and 0s that your CPU can directly execute. Try opening one up, you will just see gibberish.
The PATH
system variable stores a long list of all directories of
binary files (…/bin/) that are known by your operating system.
When you execute any program from your terminal:
my-new-cool-program
Your OS will search inside all of the directories in your
PATH
variable to find the my-new-cool-program
file.
If you are curious, you can see what is inside the PATH
system variable by running:
echo $PATH
The which
program that you executed a few lines above searches all the
places in the PATH
and tells you in which one your program resides.
In our case, python3
resides in /usr/bin/
.
Therefore, you can now execute that python3 file by running:
python3
This python3 executable file works in two different ways:
- Python Live Interpreter
You call python3 with no arguments:
python3
and it will launch the live python interpreter. This transforms your terminal into a python environement where you can type any python code.
- Python Script Execution
Or you can run the file with a single argument, the name of your .py python script:
python3 my-python-script.py
. In this case, the python3 executable will run the code in your script and then return to the terminal.
# To enter the python live interpreter
python3
# To run a python script
python3 my-python-script.py
Let’s do a hello-world example in both cases.
# Launch python live interpreter
python3
# Print "Hello World!" to the terminal
print("Hello World!")
# Exit the interpreter
CTRL-D
# Make a new .py in the current foler from the terminal
touch my-script.py
# Check it really appeared
ls
# Open it with the nano text editor
nano my-script.py
# Type in
print("Hello World!")
# Save your changes with
CTRL-o ENTER
# Exit the nano text editor with
CTRL-x
# Check the contents inside the file by printing them out
cat my-script.py
# Execute the python3 program and pass your file as an argument
python3 my-script.py
Hint
Congratulations, you now know how to write python scripts!!
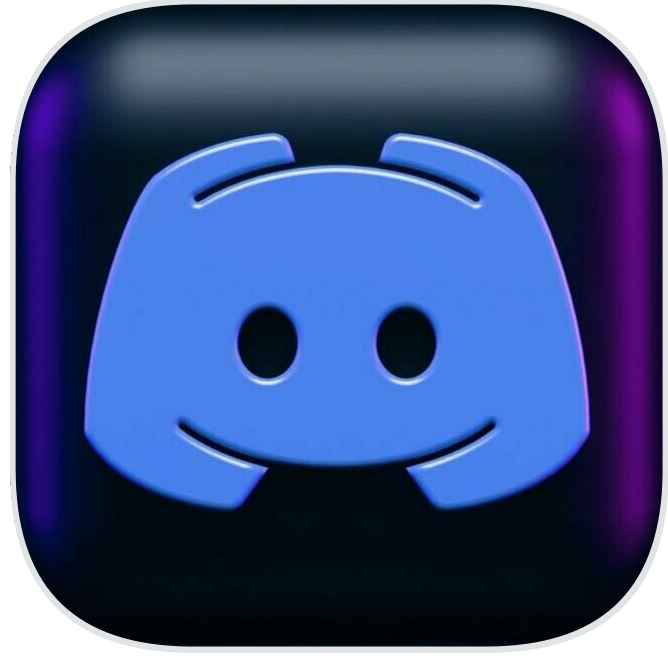
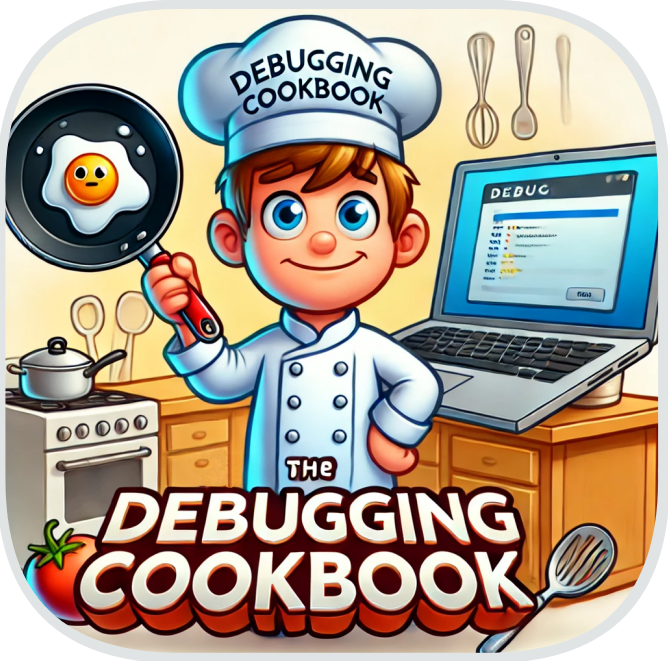
Please signal any mistake to: errata@microver.ch